Azure Functionsのサービスは、HTTPリクエストの要求を通して呼び出されるため、Azure App ServiceからAzure Functionsのサービスを呼び出すこともできる。
今回は、Azure App ServiceからAzure Functionsのサービスを呼び出してみたので、そのサンプルプログラムを共有する。
前提条件
下記記事に従ってAzure App Service, Azure Functionsのサンプルプログラムを作成済であること。
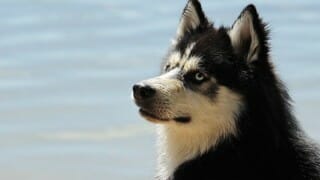
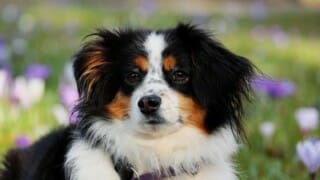
サンプルプログラムの修正
今回は、Azure App Serviceのサンプルプログラムの修正のみ行う。修正したサンプルプログラムの構成は以下の通り。
なお、上記の赤枠は、前提条件のプログラムから変更したプログラムである。
定義ファイルクラスの内容は以下の通りで、RestTemplateクラスのBean定義を作成している。
package com.example.demo; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.web.client.RestTemplate; @Configuration public class DemoConfigBean { /** * RestTemplateオブジェクトを作成する * @return RestTemplateオブジェクト */ @Bean public RestTemplate getRestTemplate(){ return new RestTemplate(); } }
また、application.propertiesの内容は以下の通りで、Azure FunctionsのサービスのベースとなるURLを設定している。
server.port = 8084 demoAzureFunc.urlBase = http://localhost:7071/api/ #demoAzureFunc.urlBase = https://azurefuncdemoapp.azurewebsites.net/api/
さらに、コントローラクラスの内容は以下の通りで、demoAzureFunctionsクラスのAPIを呼び出す処理を追加している。
package com.example.demo; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.http.HttpEntity; import org.springframework.http.HttpHeaders; import org.springframework.http.HttpMethod; import org.springframework.http.ResponseEntity; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.client.RestTemplate; @Controller public class DemoController { /** RestTemplateオブジェクト */ @Autowired private RestTemplate restTemplate; /** application.propertiesからdemoAzureFunc.urlBaseの値を取得 */ @Value("${demoAzureFunc.urlBase}") private String demoAzureFuncBase; @GetMapping("/") public String index(Model model){ // demoAzureFunctionsクラスのAPIを呼び出す HttpHeaders headers = new HttpHeaders(); HttpEntity<String> entity = new HttpEntity<String>(headers); ResponseEntity<String> response = restTemplate.exchange( demoAzureFuncBase + "hello?name=Azure" , HttpMethod.GET, entity, String.class); String resBody = response.getBody(); model.addAttribute("message", resBody); return "index"; } }
その他のソースコード内容は、以下のサイトを参照のこと。
https://github.com/purin-it/azure/tree/master/call-azure-functions-spring-java/demoAzureApp
サンプルプログラムの実行結果
サンプルプログラムの実行結果は、以下の通り。
1) ローカル環境でdemoAzureFuncアプリを「mvn azure-functions:run」コマンドで起動する。
その後、Spring Bootアプリケーションを起動し、「http:// (ホスト名):(ポート番号)」とアクセスした場合の実行結果は以下の通りで、メッセージにdemoAzureFuncアプリの呼出結果が出力される。
2) ローカル環境でdemoAzureFuncアプリのapplication.propertiesの「demoAzureFunc.urlBase」を以下のように変更する。
また、demoAzureFuncアプリにアクセスした結果は、以下のようになっているものとする。
その後、Spring Bootアプリケーションを起動し、「http:// (ホスト名):(ポート番号)」とアクセスした場合の実行結果は以下の通りで、メッセージにdemoAzureFuncアプリの呼出結果が出力される。
3) 以下のように、Azure App Service上にサンプルプログラムをデプロイする。
なお、Azure App Serviceにデプロイする過程は、以下の記事の「App ServiceへのSpring Bootを利用したJavaアプリケーションのデプロイ」を参照のこと。
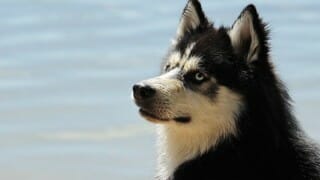
その後、Azure App ServiceのURL「https://azureappdemoservice.azurewebsites.net/」とアクセスした場合の実行結果は以下の通りで、メッセージにdemoAzureFuncアプリの呼出結果が出力される。
なお、上記URLは、下記Azure App ServiceのURLから確認できる。
要点まとめ
- Azure App ServiceからAzure Functionsを呼び出すには、REST API(Web API)を呼び出すためのメソッドを提供するRestTemplateクラスを利用すればよい。