以前、HTTPステータスが404エラーの場合に、404.htmlに遷移するようなプログラムを作成したことがあったが、今回は、HTTPステータスが404エラーの場合に、404.html以外の画面に遷移するよう修正してみたので、そのサンプルプログラムを共有する。
前提条件
下記記事の実装が完了していること。
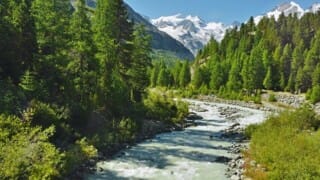
Spring Bootで特定のHTTPステータスコードが返却された場合に独自エラー画面を表示してみたSpringフレームワークでは、特定のHTTPステータスコード(例:404(Not Found))が返却された場合に独自のエラー画面に遷...
サンプルプログラムの作成
作成したサンプルプログラムの構成は以下の通り。
なお、上記の赤枠は、前提条件のプログラムから追加/変更したプログラムである。
404エラーが発生した場合に、パス「/notFoundNew」に遷移するよう設定するよう、以下のクラスを追加している。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | package com.example.demo; import org.springframework.boot.web.server.ErrorPage; import org.springframework.boot.web.server.WebServerFactoryCustomizer; import org.springframework.boot.web.servlet.server.ConfigurableServletWebServerFactory; import org.springframework.http.HttpStatus; import org.springframework.stereotype.Component; @Component public class DemoCustomConfigration implements WebServerFactoryCustomizer<ConfigurableServletWebServerFactory> { @Override public void customize(ConfigurableServletWebServerFactory factory) { // HTTP 404(NOT FOUND)エラーが発生した場合に、パス「/notFoundNew」に遷移するよう設定 factory.addErrorPages(new ErrorPage(HttpStatus.NOT_FOUND, "/notFoundNew")); } } |
また、パス「/notFoundNew」の指定を、以下のコントローラクラスに追加している。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | package com.example.demo; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestMapping; @Controller public class DemoController { /** * 初期表示画面を表示する * @return 初期表示画面 */ @GetMapping("/") public String index(){ return "index"; } /** * HTTP 500エラー(404以外)を発生させる * @return 存在しない画面 */ @PostMapping("/submit_error_other") public String submitErrorOther(){ // 存在しない画面に遷移しようとするため、 // HTTP 500エラーが発生する return "no_page"; } /** * HTTP 404エラー発生後のエラー画面に遷移させる * @return HTTP 404エラー発生後のエラー画面 */ @RequestMapping("/notFoundNew") public String notFoundNew(){ return "404new"; } } |
さらに、パス「/notFoundNew」の画面遷移先となるHTMLの内容は、以下の通り。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | <!DOCTYPE html> <html lang="ja" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>404エラー画面(新規)</title> </head> <body> <h1>404エラー画面(新規)</h1> <p> 指定されたURLが見つかりませんでした。<br/> 指定されたURLのパスがコントローラクラスに存在するかどうか確認してください。 </p> <br/><br/> <form method="get" th:action="@{/}"> <input type="submit" value="戻る" /> </form> </body> </html> |
その他のソースコード内容は、以下のサイトを参照のこと。
https://github.com/purin-it/java/tree/master/spring-boot-http-error-original/demo
サンプルプログラムの実行結果
サンプルプログラムの実行結果は、以下の通り。
1) Spring Bootアプリケーションを起動し、「http:// (ホスト名):(ポート番号)」とアクセスすると、以下のように、初期表示画面が表示されることが確認できるので、「このボタンを押すと404エラーが発生します」ボタンを押下する。
2) 以下のように、画面「404new.html」に遷移することが確認できる。
なお、変更前の遷移先画面(404.html)の表示内容は、以下の通り。
要点まとめ
- HTTPステータスが404エラーの場合に、404.html以外の画面に遷移するようにするには、ConfigurableServletWebServerFactoryインタフェースを型定義にもつ、WebServerFactoryCustomizerインタフェースを実装したクラスの、customizeメソッド内で、エラーページ設定を行えばよい。