前回、Azure Blob Storageのコンテナー内にファイルを格納するプログラムを作成したが、Azure StorageにApp ServiceやAzure Functionsからのアクセス権限を付与することで、プログラムからアクセスキーを削除することができる。
今回は、Azure StorageにApp Serviceからアクセスできる権限を追加した上で、プログラムからアクセスキーを削除してみたので、共有する。
前提条件
下記記事の実装が完了していること。
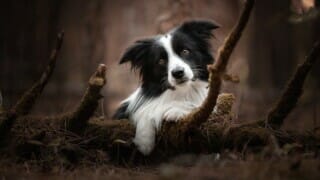
また、下記記事の「システムマネージドID割り当て(App Service)」が実施済であること。
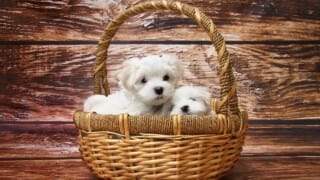
Azure Storageへのアクセス権限追加
Azure StorageにApp Serviceからアクセスできる権限を追加するのは、Azure Portal上で実施できる。その手順は、以下の通り。
1) Azure Portalにログイン後、作成したAzure Storageを表示し、「アクセス制御(IAM)」メニューを押下後、「ロールの割り当てを追加」を押下する。
2) 以下のように、ロールの割り当ての追加画面が表示されることが確認できる。
3) アクセスの割り当て先に「システム割り当てマネージドID」に表示される「App Service」を選択する。
4) 以下のように、システムマネージドIDが割り当て済のApp Serviceが表示されるので、表示された「azureAppDemoService」を選択する。
5) App ServiceからBlobストレージへの読み書きを行えるようにするため、役割に「ストレージ Blobデータ所有者」を選択する。
6) ロールの割り当て追加内容が下記のようになっていることを確認後、「保存」ボタンを押下する。
7) ロールの割り当て追加が完了すると、以下のように、右上にメッセージが表示されることが確認できる。
作成したサンプルプログラム(App Service側)の内容
作成したサンプルプログラム(App Service側)の構成は以下の通り。なお、Azure Functions側のソースコードは修正していない。
なお、上記の赤枠は、前提条件のプログラムから追加・変更したプログラムである。
pom.xmlの内容は以下の通りで、Azure Storageにアクセスするための設定を変更し、Azure認証を行うためのライブラリ(azure-identity)を追加している。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.4.0</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.example</groupId> <artifactId>demoAzureApp</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>demoAzureApp</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- lombokの設定 --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <scope>provided</scope> </dependency> <!-- Azure Storageの設定 --> <dependency> <groupId>com.azure</groupId> <artifactId>azure-storage-blob</artifactId> <version>12.10.0</version> </dependency> <dependency> <groupId>com.azure</groupId> <artifactId>azure-identity</artifactId> <version>1.2.2</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.4</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> <scope>provided</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> <plugin> <groupId>com.microsoft.azure</groupId> <artifactId>azure-webapp-maven-plugin</artifactId> <version>1.12.0</version> <configuration> <schemaVersion>v2</schemaVersion> <subscriptionId>(ログインユーザーのサブスクリプションID)</subscriptionId> <resourceGroup>azureAppDemo</resourceGroup> <appName>azureAppDemoService</appName> <pricingTier>B1</pricingTier> <region>japaneast</region> <appServicePlanName>ASP-azureAppDemo-8679</appServicePlanName> <appServicePlanResourceGroup>azureAppDemo</appServicePlanResourceGroup> <runtime> <os>Linux</os> <javaVersion>Java 8</javaVersion> <webContainer>Tomcat 8.5</webContainer> </runtime> <deployment> <resources> <resource> <directory>${project.basedir}/target</directory> <includes> <include>*.war</include> </includes> </resource> </resources> </deployment> </configuration> </plugin> </plugins> </build> </project>
application.propertiesの内容は以下の通りで、Azure Storageにアクセスするためのアクセスキーを削除している。
コントローラクラスの内容は以下の通りで、アップロードボタンが押下されたときに、Blobコンテナーにアップロードしたファイルを格納する処理を、Azure認証を行うクラス(DefaultAzureCredentialBuilder)を利用するよう修正している。
package com.example.demo; import org.apache.commons.io.IOUtils; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.multipart.MultipartFile; import org.thymeleaf.util.StringUtils; import com.azure.identity.DefaultAzureCredentialBuilder; import com.azure.storage.blob.BlobContainerClient; import com.azure.storage.blob.BlobContainerClientBuilder; import com.azure.storage.blob.specialized.BlobOutputStream; import com.azure.storage.blob.specialized.BlockBlobClient; @Controller public class DemoController { /** Azure Storageのアカウント名 */ @Value("${azure.storage.accountName}") private String storageAccountName; /** Azure StorageのBlobコンテナー名 */ @Value("${azure.storage.containerName}") private String storageContainerName; /** * メイン画面を初期表示する. * @param model Modelオブジェクト * @return メイン画面 */ @GetMapping("/") public String index(Model model) { model.addAttribute("message" , "アップロードするファイルを指定し、アップロードボタンを押下してください。"); return "main"; } /** * ファイルデータをAzure Blob Storageに登録する. * @param uploadFile アップロードファイル * @param model Modelオブジェクト * @return メイン画面 */ @PostMapping("/upload") public String add(@RequestParam("upload_file") MultipartFile uploadFile , Model model) { // ファイルが未指定の場合はエラーとする if (uploadFile == null || StringUtils.isEmptyOrWhitespace(uploadFile.getOriginalFilename())) { model.addAttribute("errMessage", "ファイルを指定してください。"); return "main"; } // ファイルアップロード処理 try { // Blob Storage内のコンテナーのクライアントオブジェクトを取得 BlobContainerClient blobContainerClient = new BlobContainerClientBuilder() .endpoint("https://" + storageAccountName + ".blob.core.windows.net") .credential(new DefaultAzureCredentialBuilder().build()) .containerName(storageContainerName) .buildClient(); // Blobコンテナー内のBlobオブジェクトを生成 BlockBlobClient blockBlobClient = blobContainerClient.getBlobClient( uploadFile.getOriginalFilename()) .getBlockBlobClient(); // Blob内のコンテナーにデータを書き込む(上書きも可能) BlobOutputStream output = blockBlobClient.getBlobOutputStream(true); output.write(IOUtils.toByteArray(uploadFile.getInputStream())); output.close(); } catch (Exception ex) { throw new RuntimeException(ex); } // メイン画面へ遷移 model.addAttribute("message", "ファイルアップロードが完了しました。"); return "main"; } }
その他のソースコード内容は、以下のサイトを参照のこと。
https://github.com/purin-it/azure/tree/master/azure-blob-storage-upload-nokey/demoAzureApp
サンプルプログラムの実行結果
サンプルプログラムの実行結果は、下記記事の実行結果と同じになる。
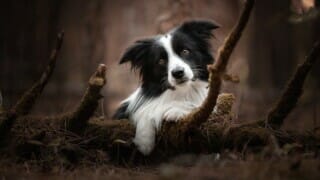
要点まとめ
- Azure StorageにApp ServiceやAzure Functionsからのアクセス権限を付与することで、Azure Blob Storageのコンテナー内にファイルを格納するプログラムからアクセスキーを削除できる。