Javaのプログラムの単体テストを自動化するツールに「Junit」があり、これを利用すると、テストを自動化でき、繰り返し実行できるようになる。
今回は、Javaの単体テストを自動化できる「Junit」の、よく使うアサーションである、org.junit.Assertクラスに含まれる「assertEquals」「assertTrue」「assertFalse」「assertNull」を使用した、作成したJunitのサンプルプログラムを共有する。
前提条件
下記記事の「IntelliJ IDEA上でSpring Bootプロジェクトの読み込み」までの手順が完了していること。
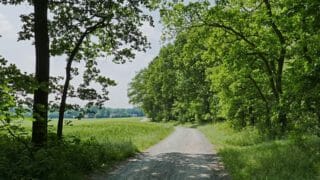
サンプルプログラムの内容
作成したサンプルプログラムの構成は以下の通り。
なお、上図の赤枠は、今回記載するサンプルプログラムの内容である。
「build.gradle」については、以下の通りで、前提条件の内容と特に変更していない。「testImplementation ‘org.springframework.boot:spring-boot-starter-test’」が入っていることにより、junitのアサーションが利用できる。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | plugins { id 'org.springframework.boot' version '2.1.7.RELEASE' id 'java' } apply plugin: 'io.spring.dependency-management' group = 'com.example' version = '0.0.1-SNAPSHOT' sourceCompatibility = '1.8' repositories { mavenCentral() } dependencies { implementation 'org.springframework.boot:spring-boot-starter-thymeleaf' implementation 'org.springframework.boot:spring-boot-starter-web' testImplementation 'org.springframework.boot:spring-boot-starter-test' } |
実際に外部ライブラリを確認すると、下図の赤枠のように、junitが含まれている。
また、テスト対象のプログラム「DemoUtil.java」の内容は以下の通り。今回は、下記クラス内のpublicメソッドをテスト対象にしている。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 | package com.example.demo; import java.time.LocalDate; import java.time.format.DateTimeFormatter; import java.time.format.ResolverStyle; public class DemoUtil { /** * 日付チェック処理を行う * @param year 年 * @param month 月 * @param day 日 * @return 判定結果(1:年が空、2:月が空、3:日が空、4:年月日が不正、0:正常 */ public static int checkDate(String year, String month, String day){ final String dateFormat = "uuuuMMdd"; if(isEmpty(year)){ return 1; } if(isEmpty(month)){ return 2; } if(isEmpty(day)){ return 3; } String dateStr = year + addZero(month) + addZero(day); if(!isCorrectDate(dateStr, dateFormat)){ return 4; } return 0; } /** * 数値文字列が1桁の場合、頭に0を付けて返す * @param intNum 数値文字列 * @return 変換後数値文字列 */ public static String addZero(String intNum){ if(isEmpty(intNum)){ return intNum; } if(intNum.length() == 1){ return "0" + intNum; } return intNum; } /** * 引数の文字列がnull、空文字かどうかを判定する * @param str チェック対象文字列 * @return 文字列チェック結果 */ public static boolean isEmpty(String str){ if(str == null || "".equals(str)){ return true; } return false; } /** * DateTimeFormatterを利用して日付チェックを行う * @param dateStr チェック対象文字列 * @param dateFormat 日付フォーマット * @return 日付チェック結果 */ private static boolean isCorrectDate(String dateStr, String dateFormat){ if(isEmpty(dateStr) || isEmpty(dateFormat)){ return false; } //日付と時刻を厳密に解決するスタイルで、DateTimeFormatterオブジェクトを作成 DateTimeFormatter df = DateTimeFormatter.ofPattern(dateFormat) .withResolverStyle(ResolverStyle.STRICT); try{ //チェック対象文字列をLocalDate型の日付に変換できれば、チェックOKとする LocalDate.parse(dateStr, df); return true; }catch(Exception e){ return false; } } } |
さらに、作成したJUnitプログラム「DemoUtilTest.java」の内容は以下の通り。テスト対象となるメソッドには、アノテーション「@Test」を付与している。org.junit.Assertクラスに含まれる「assertEquals」「assertTrue」「assertFalse」「assertNull」を使用して、テスト対象メソッドの戻り値を確認している。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 | package com.example.demo; import org.junit.Test; import static org.junit.Assert.*; public class DemoUtilTest { /** * DemoUtilクラスのcheckDateメソッドの確認 */ @Test public void testCheckDate(){ //第一引数yearがnullまたは空文字の場合に、1が返ってくることを確認 //assertEquals(期待値, 実際の値)は、期待値=実際の値であればOKと判断する int return1 = DemoUtil.checkDate(null, "12", "14"); assertEquals(1, return1); //第二引数monthがnullまたは空文字の場合に、2が返ってくることを確認 int return2 = DemoUtil.checkDate("2019", "", "14"); assertEquals(2, return2); //第三引数dayがnullまたは空文字の場合に、3が返ってくることを確認 int return3 = DemoUtil.checkDate("2019", "12", null); assertEquals(3, return3); //引数のyear,month,dayがあり得ない日付の場合に、4が返ってくることを確認 int return4 = DemoUtil.checkDate("2019", "2", "29"); assertEquals(4, return4); //引数のyear,month,dayが正しい日付の場合に、5が返ってくることを確認 int return5 = DemoUtil.checkDate("2019", "12", "14"); assertEquals(0, return5); } @Test public void testAddZero(){ //引数がnullの場合に、nullが返ってくることを確認 //assertNull(実際の値)は、実際の値=nullであればOKと判断する String return1 = DemoUtil.addZero(null); assertNull(return1); //引数が空文字の場合に、空文字が返ってくることを確認 String return2 = DemoUtil.addZero(""); assertEquals("", return2); //引数が1桁の文字列の場合に、先頭に0が付与されることを確認 String return3 = DemoUtil.addZero("1"); assertEquals("01", return3); //引数が2桁以上の文字列の場合に、引数の値が返ってくることを確認 String return4 = DemoUtil.addZero("11"); assertEquals("11", return4); } @Test public void testIsEmpty(){ //引数がnullの場合に、trueが返ってくることを確認 //assertTrue(実際の値)は、実際の値=trueであればOKと判断する boolean return1 = DemoUtil.isEmpty(null); assertTrue(return1); //引数が空文字の場合に、trueが返ってくることを確認 boolean return2 = DemoUtil.isEmpty(""); assertTrue(return2); //引数がnull・空文字以外の場合に、falseが返ってくることを確認 //assertFalse(実際の値)は、実際の値=falseであればOKと判断する boolean return3 = DemoUtil.isEmpty("abcde"); assertFalse(return3); } } |
その他のソースコード内容は、以下のサイトを参照のこと。
https://github.com/purin-it/java/tree/master/junit-assertion/demo
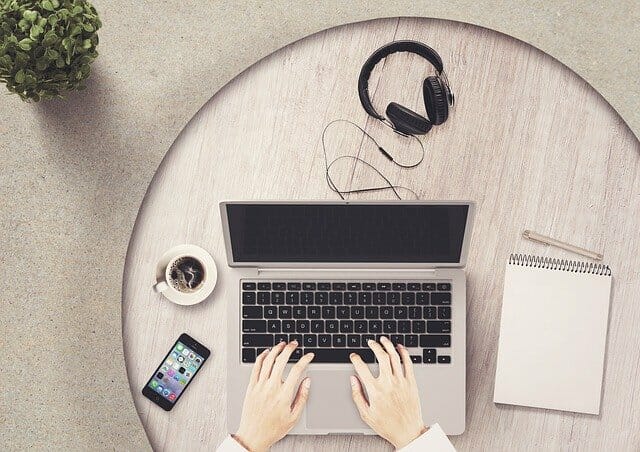
また、作成したJUnitプログラムを実行するには、下図のように、通常のJavaプログラムの実行と同じように、対象プログラムを右クリックし「実行」を選択する。
実行した結果は下図の通りで、JUnitプログラムが正常終了することが確認できた。
なお、今回取り上げた以外にも、様々なアサーションが利用できる。その内容については以下を参照のこと。
https://waman.hatenablog.com/entry/20120923/1348350852
要点まとめ
- JUnitのテスト対象となるメソッドには、アノテーション「@Test」を付与する。
- org.junit.Assertクラスに含まれる「assertEquals」「assertTrue」「assertFalse」「assertNull」等のアサーションを利用すると、想定結果と合っているかの確認が行える。