これから、ニューラルネットワークで手書き数字を分類することを考えていくが、その際に、Kerasに含まれるMNIST(手描き数字)のデータを利用することができる。
今回は、Kerasに含まれるMNIST(手描き数字)のデータを読み込み、中身を確認してみたので、そのサンプルプログラムを共有する。
Kerasに含まれるMNIST(手描き数字)のデータを読み込んだ結果は以下の通りで、訓練用データとテスト用データを別々に取得することができる。
from tensorflow.keras.datasets import mnist # Kerasに含まれているMNIST(手描き数字)のデータを読み込む (x_train, y_train), (x_test, y_test) = mnist.load_data() # 読み込んだデータの形状を確認 print("*** x_train(訓練用データ(入力データ))の形状 ***") print(x_train.shape) print("*** y_train(訓練用データ(正解データ))の形状 ***") print(y_train.shape) print() print("*** x_test(テスト用データ(入力データ))の形状 ***") print(x_test.shape) print("*** y_test(テスト用データ(正解データ))の形状 ***") print(y_test.shape)
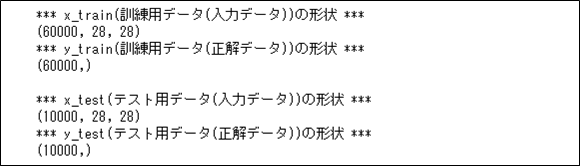
また、Kerasに含まれるMNIST(手描き数字)のデータを確認した結果は以下の通りで、入力データと正解データの内容が一致していることが確認できる。
%matplotlib inline from tensorflow.keras.datasets import mnist import matplotlib.pyplot as plt # Kerasに含まれているMNIST(手描き数字)のデータを読み込む (x_train, y_train), (x_test, y_test) = mnist.load_data() # 読み込んだ訓練用データを表示 print("*** y_train(訓練用データ(正解データ))の先頭5件のデータ ***") print(y_train[0:5]) print() print("*** x_train(訓練用データ(入力データ))の先頭5件のデータ ***") for i in range(5): plt.subplot(1, 5, i+1) plt.title("Label: " + str(i)) plt.imshow(x_train[i].reshape(28,28), cmap=None) plt.show()
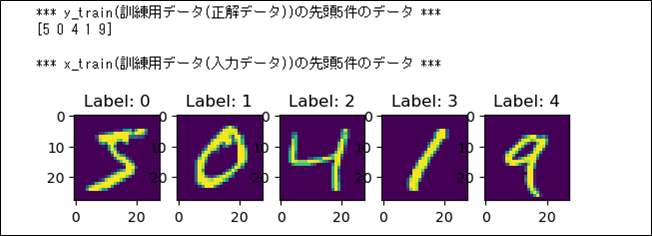
%matplotlib inline from tensorflow.keras.datasets import mnist import matplotlib.pyplot as plt # Kerasに含まれているMNIST(手描き数字)のデータを読み込む (x_train, y_train), (x_test, y_test) = mnist.load_data() # 読み込んだテスト用データを表示 print("*** y_test(テスト用データ(正解データ))の先頭5件のデータ ***") print(y_test[0:5]) print() print("*** x_test(テスト用データ(入力データ))の先頭5件のデータ ***") for i in range(5): plt.subplot(1, 5, i+1) plt.title("Label: " + str(i)) plt.imshow(x_test[i].reshape(28,28), cmap=None) plt.show()
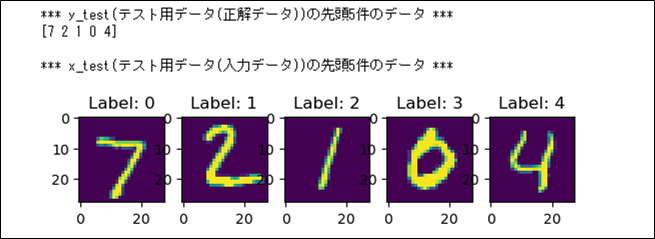
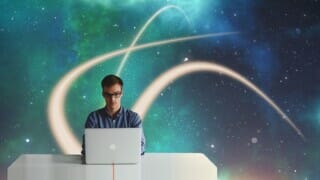
サラリーマン型フリーランスSEという働き方でお金の不安を解消しよう先日、「サラリーマン型フリーランスSE」という働き方を紹介するYouTube動画を視聴しましたので、その内容をご紹介します。 「サ...
さらに、Kerasに含まれるMNIST(手描き数字)の入力データを1件確認した結果は、以下の通り。
from tensorflow.keras.datasets import mnist # Kerasに含まれているMNIST(手描き数字)のデータを読み込む (x_train, y_train), (x_test, y_test) = mnist.load_data() # 読み込んだ訓練用データ(入力データ)の1件目を表示 print("*** x_train(訓練用データ(入力データ))の1件目のデータ ***") print(x_train[0])
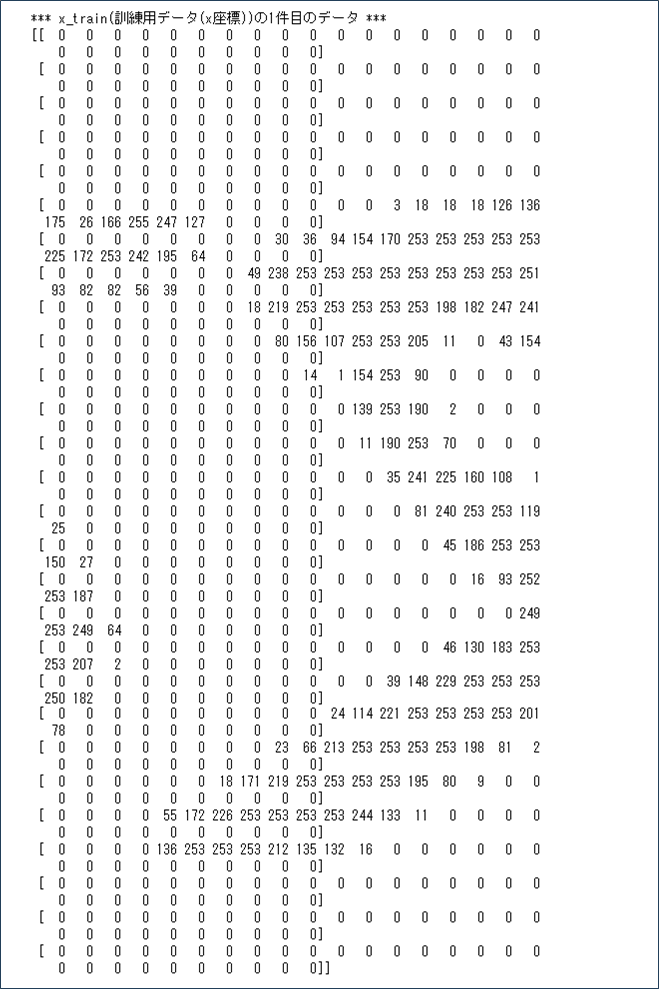
なお、上記MNIST(手描き数字)の入力データの説明は、以下のサイトを参照のこと。
https://atmarkit.itmedia.co.jp/ait/articles/2001/22/news012.html
また、ディープラーニングでKerasに含まれるMNIST(手描き数字)の入力データを利用する際、以下のように、入力データを0~1の範囲に変換して利用する。
from tensorflow.keras.datasets import mnist import numpy as np # Kerasに含まれているMNIST(手描き数字)のデータを読み込む (x_train, y_train), (x_test, y_test) = mnist.load_data() # 読み込んだ訓練用データ(入力データ)の1件目を255で除算した値を表示 print("*** x_train(訓練用データ(入力データ))の1件目の変換後データ ***") np.set_printoptions(precision=2, suppress=True) print(x_train[0]/255.0)
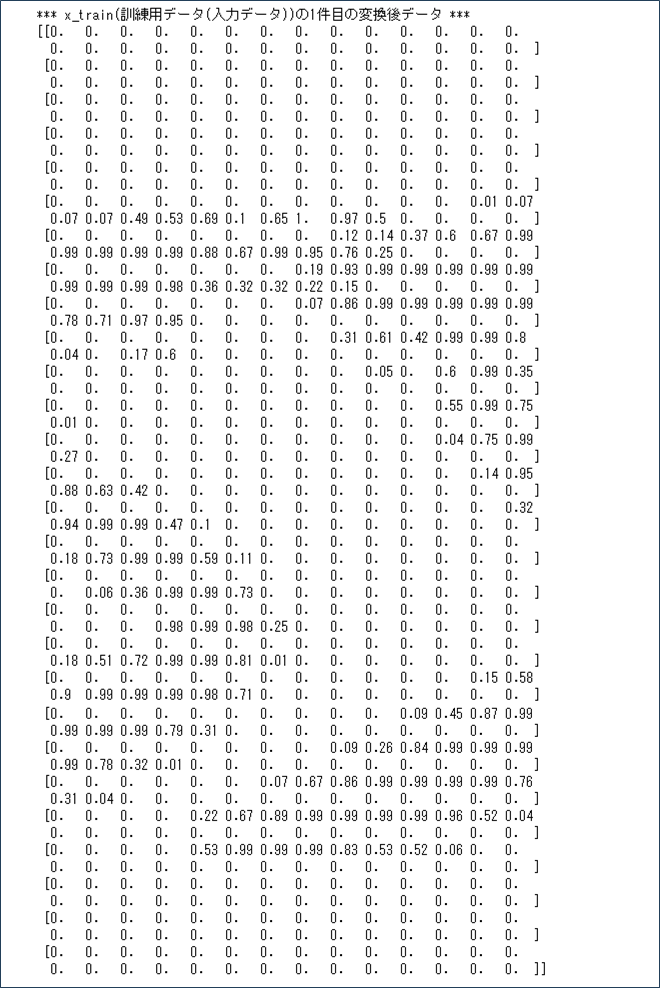
さらに、ディープラーニングでKerasに含まれるMNIST(手描き数字)の正解データを利用する際、以下のように、正解データをOne-Hotエンコーディング変換して利用する。
from tensorflow.keras.datasets import mnist from tensorflow.keras.utils import to_categorical # Kerasに含まれているMNISTのデータを読み込む (x_train, y_train), (x_test, y_test) = mnist.load_data() # 読み込んだ訓練用データ(y座標)の先頭5件を表示 print("*** y_train(訓練用データ(正解データ))の先頭5件のデータ ***") print(y_train[0:5]) print() # 読み込んだ訓練用データ(y座標)の先頭5件を # One-Hotエンコーディング変換した値を表示 print("*** y_train(訓練用データ(正解データ))の先頭5件の変換後データ ***") print(to_categorical(y_train[0:5],10))
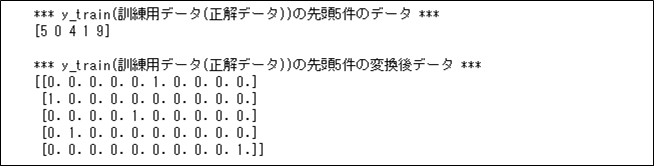
要点まとめ
- ニューラルネットワークで手書き数字を分類することを考える際、Kerasに含まれるMNIST(手描き数字)のデータを利用できる。