SpringフレームワークでMyBatisを利用する際、EntityクラスやMapperクラス、Mapperクラスが参照するXMLファイルが必要になってくるが、それらはMyBatis Generatorによって自動生成することができる。
今回は、MyBatis GeneratorによってEntityクラスやMapperクラス、Mapperクラスが参照するXMLファイルを自動生成してみたので、その手順を共有する。
なお、Entityクラスの例は、以下のサイトの「UserData.java」を参照のこと。
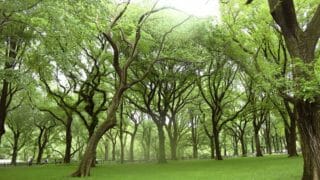
また、MapperクラスやMapperクラスが参照するXMLファイルの例は、以下のサイトを参照のこと。
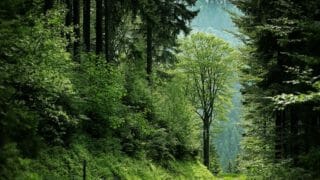
前提条件
下記記事の手順に従って、STSをダウンロード済であること。
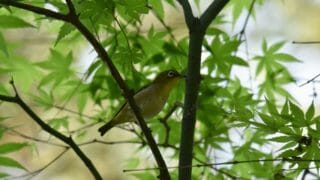
また、データベース上に以下のテーブルが作成されていること。
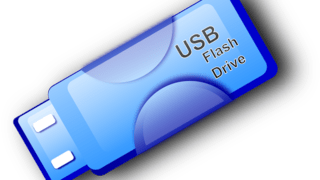
やってみたこと
MyBatis Generatorのインストール
MyBatis Generatorのインストール手順は、以下の通り。
1) STSを起動し、「ヘルプ」メニューから「Eclipse マーケットプレース」を選択する。
2) 検索ウィンドウに「MyBatis Generator」を指定し、「Go」ボタンを押下する。
3) 「MyBatis Generator」の「インストール」ボタンを押下する。
4) ライセンスのレビュー画面が表示されるので、「使用条件の条項に同意します」を選択後、「完了」ボタンを押下する。
5) セキュリティー警告ダイアログが表示されるので、「インストール」ボタンを押下する。
6) ソフトウェア更新ダイアログが表示されるので、「今すぐ再起動」ボタンを押下する。
7) パッケージ・エクスプローラー上で右クリックし、「新規」メニューから「その他」を選択すると、以下のように、「MyBatis Generator Configuration File」メニューが確認できる。
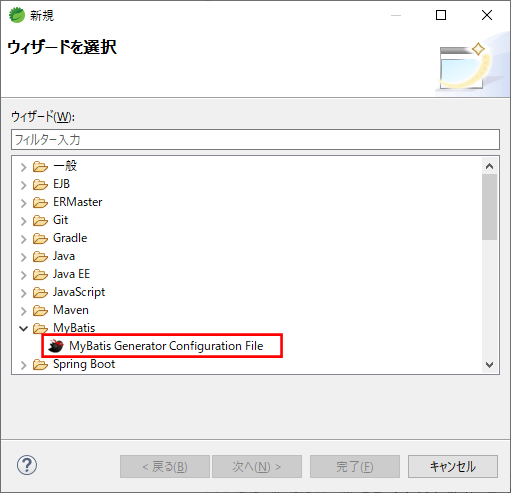
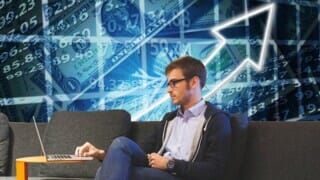
Spring Bootプロジェクトの作成
STS上で、MyBatis GeneratorによってEntityクラスやMapperクラス、Mapperクラスが参照するXMLファイルを自動生成の対象とするSpring Bootプロジェクトを作成する。その手順は以下の通り。
1) パッケージ・エクスプローラー上で右クリックし、「新規」メニューから「Spring スターター・プロジェクト」を選択する。
2) 以下のように作成するプロジェクトの情報を指定し、「次へ」ボタンを押下する。
3) MyBatis Frameworkのチェックを指定し、「次へ」ボタンを押下する。
4) 以下の画面が表示されるので、「完了」ボタンを押下する。なお、下記画面では環境の都合上、Javaのバージョンを13に変更している。
5) 以下のように、「demo2」プロジェクトが作成されることが確認できる。
6) Oracleにojdbc経由で接続するため、以下のように、libフォルダ下にJDBCドライバ「ojdbc6.jar」を配置する。
7) Oracleにojdbc経由で接続するため、pom.xmlに以下の記述を追加する。
<!-- oracleを利用するための設定 --> <dependency> <groupId>com.oracle.jdbc</groupId> <artifactId>ojdbc6</artifactId> <version>1.0</version> <scope>system</scope> <systemPath>${basedir}/lib/ojdbc6.jar</systemPath> </dependency>
追加後のpom.xmlの内容は以下の通り。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.3.4.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.example</groupId> <artifactId>demo2</artifactId> <version>0.0.1-SNAPSHOT</version> <name>demo2</name> <description>Demo project for Spring Boot</description> <properties> <java.version>13</java.version> </properties> <dependencies> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>2.1.3</version> </dependency> <!-- oracleを利用するための設定 --> <dependency> <groupId>com.oracle.jdbc</groupId> <artifactId>ojdbc6</artifactId> <version>1.0</version> <scope>system</scope> <systemPath>${basedir}/lib/ojdbc6.jar</systemPath> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
8) 今回作成したプロジェクトを選択し右クリックし、「Maven」から「プロジェクトの更新」を選択する。
以下のように、Maven更新対象のプロジェクトが選択された状態で、「OK」ボタンを押下する。
MyBatis Generator用の設定ファイルの作成
MyBatis Generator用の設定ファイルの作成手順は、以下の通り。
1) 作成したプロジェクトの「src/main/resources」フォルダを選択し右クリックし、「新規」メニューから「その他」を選択する。
2) 「MyBatis Generator Configuration File」を選択し、「次へ」ボタンを押下する。
3) 以下の画面が表示されるので、「完了」ボタンを押下する。
4) 以下のように、「generatorConfig.xml」が作成されることが確認できる。
5) 作成された「generatorConfig.xml」を、以下の内容に変更する。
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE generatorConfiguration PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN" "http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd"> <generatorConfiguration> <context id="OracleTables" targetRuntime="MyBatis3"> <!-- 参照元となるテーブルのあるDBへの接続設定 --> <jdbcConnection driverClass="oracle.jdbc.driver.OracleDriver" connectionURL="jdbc:oracle:thin:@localhost:1521:xe" userId="USER01" password="USER01"> </jdbcConnection> <!-- UserDataクラスを生成する設定 --> <javaModelGenerator targetPackage="com.example.demo" targetProject="demo2/src/main/java"> <property name="enableSubPackages" value="true" /> <property name="trimStrings" value="true" /> </javaModelGenerator> <!-- UserDataMapperのXMLファイルを生成する設定 --> <sqlMapGenerator targetPackage="com.example.demo" targetProject="demo2/src/main/resources"> <property name="enableSubPackages" value="true" /> </sqlMapGenerator> <!-- UserDataMapperクラスを生成する設定 --> <javaClientGenerator type="XMLMAPPER" targetPackage="com.example.demo" targetProject="demo2/src/main/java"> <property name="enableSubPackages" value="true" /> </javaClientGenerator> <!-- 参照元となるテーブルを指定 --> <table tableName="user_data"> <property name="useActualColumnNames" value="true" /> </table> </context> </generatorConfiguration>
MyBatis Generatorの実行
MyBatis Generatorの実行手順は、以下の通り。
1) 「generatorConfig.xml」を選択し右クリックし、「実行」から「Run MyBatis Generator」を指定する。
3) また、実行した結果、以下の赤枠のファイルが作成される。
作成された「UserData.java」の内容は、以下の通り。
package com.example.demo; public class UserData { /** * This field was generated by MyBatis Generator. This field corresponds to the database column USER_DATA.ID * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ private Integer ID; /** * This field was generated by MyBatis Generator. This field corresponds to the database column USER_DATA.NAME * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ private String NAME; /** * This field was generated by MyBatis Generator. This field corresponds to the database column USER_DATA.BIRTH_YEAR * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ private Short BIRTH_YEAR; /** * This field was generated by MyBatis Generator. This field corresponds to the database column USER_DATA.BIRTH_MONTH * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ private Short BIRTH_MONTH; /** * This field was generated by MyBatis Generator. This field corresponds to the database column USER_DATA.BIRTH_DAY * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ private Short BIRTH_DAY; /** * This field was generated by MyBatis Generator. This field corresponds to the database column USER_DATA.SEX * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ private String SEX; /** * This field was generated by MyBatis Generator. This field corresponds to the database column USER_DATA.MEMO * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ private String MEMO; /** * This method was generated by MyBatis Generator. This method returns the value of the database column USER_DATA.ID * @return the value of USER_DATA.ID * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public Integer getID() { return ID; } /** * This method was generated by MyBatis Generator. This method sets the value of the database column USER_DATA.ID * @param ID the value for USER_DATA.ID * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public void setID(Integer ID) { this.ID = ID; } /** * This method was generated by MyBatis Generator. This method returns the value of the database column USER_DATA.NAME * @return the value of USER_DATA.NAME * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public String getNAME() { return NAME; } /** * This method was generated by MyBatis Generator. This method sets the value of the database column USER_DATA.NAME * @param NAME the value for USER_DATA.NAME * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public void setNAME(String NAME) { this.NAME = NAME == null ? null : NAME.trim(); } /** * This method was generated by MyBatis Generator. This method returns the value of the database column USER_DATA.BIRTH_YEAR * @return the value of USER_DATA.BIRTH_YEAR * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public Short getBIRTH_YEAR() { return BIRTH_YEAR; } /** * This method was generated by MyBatis Generator. This method sets the value of the database column USER_DATA.BIRTH_YEAR * @param BIRTH_YEAR the value for USER_DATA.BIRTH_YEAR * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public void setBIRTH_YEAR(Short BIRTH_YEAR) { this.BIRTH_YEAR = BIRTH_YEAR; } /** * This method was generated by MyBatis Generator. This method returns the value of the database column USER_DATA.BIRTH_MONTH * @return the value of USER_DATA.BIRTH_MONTH * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public Short getBIRTH_MONTH() { return BIRTH_MONTH; } /** * This method was generated by MyBatis Generator. This method sets the value of the database column USER_DATA.BIRTH_MONTH * @param BIRTH_MONTH the value for USER_DATA.BIRTH_MONTH * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public void setBIRTH_MONTH(Short BIRTH_MONTH) { this.BIRTH_MONTH = BIRTH_MONTH; } /** * This method was generated by MyBatis Generator. This method returns the value of the database column USER_DATA.BIRTH_DAY * @return the value of USER_DATA.BIRTH_DAY * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public Short getBIRTH_DAY() { return BIRTH_DAY; } /** * This method was generated by MyBatis Generator. This method sets the value of the database column USER_DATA.BIRTH_DAY * @param BIRTH_DAY the value for USER_DATA.BIRTH_DAY * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public void setBIRTH_DAY(Short BIRTH_DAY) { this.BIRTH_DAY = BIRTH_DAY; } /** * This method was generated by MyBatis Generator. This method returns the value of the database column USER_DATA.SEX * @return the value of USER_DATA.SEX * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public String getSEX() { return SEX; } /** * This method was generated by MyBatis Generator. This method sets the value of the database column USER_DATA.SEX * @param SEX the value for USER_DATA.SEX * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public void setSEX(String SEX) { this.SEX = SEX == null ? null : SEX.trim(); } /** * This method was generated by MyBatis Generator. This method returns the value of the database column USER_DATA.MEMO * @return the value of USER_DATA.MEMO * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public String getMEMO() { return MEMO; } /** * This method was generated by MyBatis Generator. This method sets the value of the database column USER_DATA.MEMO * @param MEMO the value for USER_DATA.MEMO * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ public void setMEMO(String MEMO) { this.MEMO = MEMO == null ? null : MEMO.trim(); } }
また、作成された「UserDataMapper.java」の内容は、以下の通り。
package com.example.demo; import com.example.demo.UserData; import com.example.demo.UserDataExample; import java.util.List; import org.apache.ibatis.annotations.Param; public interface UserDataMapper { /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ long countByExample(UserDataExample example); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ int deleteByExample(UserDataExample example); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ int deleteByPrimaryKey(Integer ID); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ int insert(UserData record); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ int insertSelective(UserData record); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ List<UserData> selectByExample(UserDataExample example); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ UserData selectByPrimaryKey(Integer ID); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ int updateByExampleSelective(@Param("record") UserData record, @Param("example") UserDataExample example); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ int updateByExample(@Param("record") UserData record, @Param("example") UserDataExample example); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ int updateByPrimaryKeySelective(UserData record); /** * This method was generated by MyBatis Generator. This method corresponds to the database table USER_DATA * @mbg.generated Fri Oct 02 18:49:46 JST 2020 */ int updateByPrimaryKey(UserData record); }
さらに、作成された「UserDataMapper.xml」の内容は、以下の通り。
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.example.demo.UserDataMapper"> <resultMap id="BaseResultMap" type="com.example.demo.UserData"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> <id column="ID" jdbcType="DECIMAL" property="ID" /> <result column="NAME" jdbcType="VARCHAR" property="NAME" /> <result column="BIRTH_YEAR" jdbcType="DECIMAL" property="BIRTH_YEAR" /> <result column="BIRTH_MONTH" jdbcType="DECIMAL" property="BIRTH_MONTH" /> <result column="BIRTH_DAY" jdbcType="DECIMAL" property="BIRTH_DAY" /> <result column="SEX" jdbcType="CHAR" property="SEX" /> <result column="MEMO" jdbcType="VARCHAR" property="MEMO" /> </resultMap> <sql id="Example_Where_Clause"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> <where> <foreach collection="oredCriteria" item="criteria" separator="or"> <if test="criteria.valid"> <trim prefix="(" prefixOverrides="and" suffix=")"> <foreach collection="criteria.criteria" item="criterion"> <choose> <when test="criterion.noValue"> and ${criterion.condition} </when> <when test="criterion.singleValue"> and ${criterion.condition} #{criterion.value} </when> <when test="criterion.betweenValue"> and ${criterion.condition} #{criterion.value} and #{criterion.secondValue} </when> <when test="criterion.listValue"> and ${criterion.condition} <foreach close=")" collection="criterion.value" item="listItem" open="(" separator=","> #{listItem} </foreach> </when> </choose> </foreach> </trim> </if> </foreach> </where> </sql> <sql id="Update_By_Example_Where_Clause"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> <where> <foreach collection="example.oredCriteria" item="criteria" separator="or"> <if test="criteria.valid"> <trim prefix="(" prefixOverrides="and" suffix=")"> <foreach collection="criteria.criteria" item="criterion"> <choose> <when test="criterion.noValue"> and ${criterion.condition} </when> <when test="criterion.singleValue"> and ${criterion.condition} #{criterion.value} </when> <when test="criterion.betweenValue"> and ${criterion.condition} #{criterion.value} and #{criterion.secondValue} </when> <when test="criterion.listValue"> and ${criterion.condition} <foreach close=")" collection="criterion.value" item="listItem" open="(" separator=","> #{listItem} </foreach> </when> </choose> </foreach> </trim> </if> </foreach> </where> </sql> <sql id="Base_Column_List"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> ID, NAME, BIRTH_YEAR, BIRTH_MONTH, BIRTH_DAY, SEX, MEMO </sql> <select id="selectByExample" parameterType="com.example.demo.UserDataExample" resultMap="BaseResultMap"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> select <if test="distinct"> distinct </if> <include refid="Base_Column_List" /> from USER_DATA <if test="_parameter != null"> <include refid="Example_Where_Clause" /> </if> <if test="orderByClause != null"> order by ${orderByClause} </if> </select> <select id="selectByPrimaryKey" parameterType="java.lang.Integer" resultMap="BaseResultMap"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> select <include refid="Base_Column_List" /> from USER_DATA where ID = #{ID,jdbcType=DECIMAL} </select> <delete id="deleteByPrimaryKey" parameterType="java.lang.Integer"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> delete from USER_DATA where ID = #{ID,jdbcType=DECIMAL} </delete> <delete id="deleteByExample" parameterType="com.example.demo.UserDataExample"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> delete from USER_DATA <if test="_parameter != null"> <include refid="Example_Where_Clause" /> </if> </delete> <insert id="insert" parameterType="com.example.demo.UserData"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> insert into USER_DATA (ID, NAME, BIRTH_YEAR, BIRTH_MONTH, BIRTH_DAY, SEX, MEMO) values (#{ID,jdbcType=DECIMAL}, #{NAME,jdbcType=VARCHAR}, #{BIRTH_YEAR,jdbcType=DECIMAL}, #{BIRTH_MONTH,jdbcType=DECIMAL}, #{BIRTH_DAY,jdbcType=DECIMAL}, #{SEX,jdbcType=CHAR}, #{MEMO,jdbcType=VARCHAR}) </insert> <insert id="insertSelective" parameterType="com.example.demo.UserData"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> insert into USER_DATA <trim prefix="(" suffix=")" suffixOverrides=","> <if test="ID != null"> ID, </if> <if test="NAME != null"> NAME, </if> <if test="BIRTH_YEAR != null"> BIRTH_YEAR, </if> <if test="BIRTH_MONTH != null"> BIRTH_MONTH, </if> <if test="BIRTH_DAY != null"> BIRTH_DAY, </if> <if test="SEX != null"> SEX, </if> <if test="MEMO != null"> MEMO, </if> </trim> <trim prefix="values (" suffix=")" suffixOverrides=","> <if test="ID != null"> #{ID,jdbcType=DECIMAL}, </if> <if test="NAME != null"> #{NAME,jdbcType=VARCHAR}, </if> <if test="BIRTH_YEAR != null"> #{BIRTH_YEAR,jdbcType=DECIMAL}, </if> <if test="BIRTH_MONTH != null"> #{BIRTH_MONTH,jdbcType=DECIMAL}, </if> <if test="BIRTH_DAY != null"> #{BIRTH_DAY,jdbcType=DECIMAL}, </if> <if test="SEX != null"> #{SEX,jdbcType=CHAR}, </if> <if test="MEMO != null"> #{MEMO,jdbcType=VARCHAR}, </if> </trim> </insert> <select id="countByExample" parameterType="com.example.demo.UserDataExample" resultType="java.lang.Long"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> select count(*) from USER_DATA <if test="_parameter != null"> <include refid="Example_Where_Clause" /> </if> </select> <update id="updateByExampleSelective" parameterType="map"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> update USER_DATA <set> <if test="record.ID != null"> ID = #{record.ID,jdbcType=DECIMAL}, </if> <if test="record.NAME != null"> NAME = #{record.NAME,jdbcType=VARCHAR}, </if> <if test="record.BIRTH_YEAR != null"> BIRTH_YEAR = #{record.BIRTH_YEAR,jdbcType=DECIMAL}, </if> <if test="record.BIRTH_MONTH != null"> BIRTH_MONTH = #{record.BIRTH_MONTH,jdbcType=DECIMAL}, </if> <if test="record.BIRTH_DAY != null"> BIRTH_DAY = #{record.BIRTH_DAY,jdbcType=DECIMAL}, </if> <if test="record.SEX != null"> SEX = #{record.SEX,jdbcType=CHAR}, </if> <if test="record.MEMO != null"> MEMO = #{record.MEMO,jdbcType=VARCHAR}, </if> </set> <if test="_parameter != null"> <include refid="Update_By_Example_Where_Clause" /> </if> </update> <update id="updateByExample" parameterType="map"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> update USER_DATA set ID = #{record.ID,jdbcType=DECIMAL}, NAME = #{record.NAME,jdbcType=VARCHAR}, BIRTH_YEAR = #{record.BIRTH_YEAR,jdbcType=DECIMAL}, BIRTH_MONTH = #{record.BIRTH_MONTH,jdbcType=DECIMAL}, BIRTH_DAY = #{record.BIRTH_DAY,jdbcType=DECIMAL}, SEX = #{record.SEX,jdbcType=CHAR}, MEMO = #{record.MEMO,jdbcType=VARCHAR} <if test="_parameter != null"> <include refid="Update_By_Example_Where_Clause" /> </if> </update> <update id="updateByPrimaryKeySelective" parameterType="com.example.demo.UserData"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> update USER_DATA <set> <if test="NAME != null"> NAME = #{NAME,jdbcType=VARCHAR}, </if> <if test="BIRTH_YEAR != null"> BIRTH_YEAR = #{BIRTH_YEAR,jdbcType=DECIMAL}, </if> <if test="BIRTH_MONTH != null"> BIRTH_MONTH = #{BIRTH_MONTH,jdbcType=DECIMAL}, </if> <if test="BIRTH_DAY != null"> BIRTH_DAY = #{BIRTH_DAY,jdbcType=DECIMAL}, </if> <if test="SEX != null"> SEX = #{SEX,jdbcType=CHAR}, </if> <if test="MEMO != null"> MEMO = #{MEMO,jdbcType=VARCHAR}, </if> </set> where ID = #{ID,jdbcType=DECIMAL} </update> <update id="updateByPrimaryKey" parameterType="com.example.demo.UserData"> <!-- WARNING - @mbg.generated This element is automatically generated by MyBatis Generator, do not modify. This element was generated on Fri Oct 02 18:49:46 JST 2020. --> update USER_DATA set NAME = #{NAME,jdbcType=VARCHAR}, BIRTH_YEAR = #{BIRTH_YEAR,jdbcType=DECIMAL}, BIRTH_MONTH = #{BIRTH_MONTH,jdbcType=DECIMAL}, BIRTH_DAY = #{BIRTH_DAY,jdbcType=DECIMAL}, SEX = #{SEX,jdbcType=CHAR}, MEMO = #{MEMO,jdbcType=VARCHAR} where ID = #{ID,jdbcType=DECIMAL} </update> </mapper>
要点まとめ
- MyBatis Generatorを利用すると、SpringフレームワークでMyBatisを利用する際に必要な、EntityクラスやMapperクラス、Mapperクラスが参照するXMLファイルを自動生成できる。
- STS上でMyBatis Generatorを利用するには、MyBatis GeneratorをEclipse マーケットプレースからインストールし、定義ファイル「generatorConfig.xml」を作成後、実行すればよい。