前回は、クライアント側からサーバー側で、REST API接続によりユーザーデータを取得しクライアント側の画面に表示していたが、今回は、REST API接続によりユーザーデータのリストを取得しクライアント側の画面に表示するサンプルプログラムを作成してみたので、共有する。
前提条件
下記記事のREST API接続するサンプルプログラムの作成が完了していること。
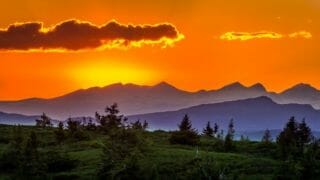
また、Oracle上でuser_dataテーブルを作成し、以下のデータが作成できていること。
サーバー側のプログラムの作成と実行
作成したサーバー側(demo_server)サンプルプログラムの構成は以下の通り。
なお、上記の赤枠は、前提条件のプログラムから変更したプログラムである。
コントローラクラスの内容は以下の通りで、ユーザーデータリストを取得し、性別を表示用(男,女)に変換し、エンコードするメソッド(getUserDataList)を用意している。
package com.example.demo; import com.fasterxml.jackson.core.JsonProcessingException; import com.fasterxml.jackson.databind.ObjectMapper; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.domain.Sort; import org.springframework.util.StringUtils; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; import java.io.UnsupportedEncodingException; import java.net.URLEncoder; import java.util.List; import static org.springframework.data.domain.Sort.Direction.ASC; @RestController public class DemoController { /** * ユーザーデータテーブル(user_data)へアクセスするリポジトリ */ @Autowired private UserDataRepository repository; /** * ユーザーデータを全件取得する * @return ユーザーデータリスト(JSON形式) */ @GetMapping("/getUserDataList") public String getUserDataList(){ List<UserData> userDataList = repository.findAll(new Sort(ASC, "id")); // ユーザーデータが取得できなかった場合は、null値を返す if(userDataList == null || userDataList.size() == 0){ return null; } for(UserData userData : userDataList){ // 性別を表示用(男,女)に変換 userData.setSex("1".equals(userData.getSex()) ? "男" : "女"); // 名前・メモ・性別をエンコード userData.setName(encode(userData.getName())); userData.setMemo(encode(userData.getMemo())); userData.setSex(encode(userData.getSex())); } // 取得したユーザーデータをJSON文字列に変換し返却 return getJsonData(userDataList); } /** * 引数の文字列をエンコードする * @param data 任意の文字列 * @return エンコード後の文字列 */ private String encode(String data){ if(StringUtils.isEmpty(data)){ return data; } String retVal = null; try{ retVal = URLEncoder.encode(data, "UTF-8"); }catch (UnsupportedEncodingException e) { System.err.println(e); } return retVal; } /** * 引数のオブジェクトをJSON文字列に変換する * @param data オブジェクトのデータ * @return 変換後JSON文字列 */ private String getJsonData(Object data){ String retVal = null; ObjectMapper objectMapper = new ObjectMapper(); try{ retVal = objectMapper.writeValueAsString(data); } catch (JsonProcessingException e) { System.err.println(e); } return retVal; } }
その他のソースコード内容は、以下のサイトを参照のこと。
https://github.com/purin-it/java/tree/master/spring-boot-rest-api-list/demo_server
上記プログラムを作成し、Spring Bootアプリケーションを起動し、「http://localhost:8085/getUserDataList」にアクセスした結果は以下の通り。
文字エンコードを実施しているため、日本語の文字はエンコードされているが、user_dataテーブルの全データがJSON形式で表示されることが確認できる。
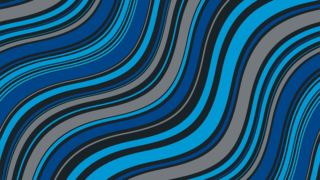
クライアント側のプログラムの作成と実行
作成したクライアント側(demo)サンプルプログラムの構成は以下の通り。
なお、上記の赤枠は、前提条件のプログラムから変更したプログラムである。
コントローラクラスの内容は以下の通り。
package com.example.demo; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.core.ParameterizedTypeReference; import org.springframework.http.HttpMethod; import org.springframework.http.ResponseEntity; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.util.StringUtils; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.client.RestTemplate; import java.io.UnsupportedEncodingException; import java.net.URLDecoder; import java.util.List; @Controller public class DemoController { /** * RestTemplateオブジェクト */ @Autowired private RestTemplate restTemplate; /** * ユーザーデータを取得し、初期表示画面に遷移する * @param model Modelオブジェクト * @return 初期表示画面へのパス */ @GetMapping("/") public String index(Model model){ // ユーザーデータリストをAPIで取得する ResponseEntity<List<UserData>> response = restTemplate.exchange( "http://localhost:8085/getUserDataList", HttpMethod.GET, null, new ParameterizedTypeReference<List<UserData>>() {}); List<UserData> userDataList = response.getBody(); // 各ユーザーデータを編集し、Modelに設定する for(UserData userData : userDataList){ // 名前・メモ・性別をデコード userData.setName(decode(userData.getName())); userData.setMemo(decode(userData.getMemo())); userData.setSex(decode(userData.getSex())); } model.addAttribute("userDataList", userDataList); return "index"; } /** * 引数の文字列をデコードする * @param data 任意の文字列 * @return エンコード後の文字列 */ private String decode(String data){ if(StringUtils.isEmpty(data)){ return data; } String retVal = null; try{ retVal = URLDecoder.decode(data, "UTF-8"); }catch (UnsupportedEncodingException e) { System.err.println(e); } return retVal; } }
また、index.htmlの内容は以下の通りで、初期表示画面を定義し、user_dataテーブルの全データの値を画面上に表示している。
<!DOCTYPE html> <html lang="ja" xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8"> <title>index page</title> </head> <body> ★以下に検索されたデータが表示されます <table border="1" cellpadding="5"> <tr> <th>ID</th> <th>名前</th> <th>生年月日</th> <th>性別</th> <th>メモ</th> </tr> <tr th:each="obj : ${userDataList}"> <td th:text="${obj.id}"></td> <td th:text="${obj.name}"></td> <td th:text="|${obj.birthY}年 ${obj.birthM}月 ${obj.birthD}日|"></td> <td th:text="${obj.sex}"></td> <td th:text="${obj.memo}"></td> </tr> </table> </body> </html>
その他のソースコード内容は、以下のサイトを参照のこと。
https://github.com/purin-it/java/tree/master/spring-boot-rest-api-list/demo
https://www.purin-it.com/doctor-homenet
サーバー・クライアント両方のSpring Bootアプリケーションを起動し、「http:// (ホスト名):8084」とアクセスすると、以下の画面が表示されることが確認できる。
要点まとめ
- API接続を行って、リストのJSON文字列からUserDataオブジェクトを取得するには、RestTemplateクラスのexchangeメソッドを利用し、第一引数にURL、第二引数にHttpMethod.GET、第四引数にリスト型のParameterizedTypeReferenceクラスのインスタンスを指定すればよい。