Spring Securityには、セキュリティ対策の基本機能として「認証」「認可」機能が含まれる。詳細は以下のサイトを参照のこと。
https://terasolunaorg.github.io/guideline/5.1.0.RELEASE/ja/Security/SpringSecurity.html#springsecurityfunctionalities
今回は、Spring Securityを利用して、ユーザー名・パスワードによる認証を行う「Basic認証」を行うサンプルプログラムを作成してみたので、共有する。
前提条件
下記記事の実装が完了していること。
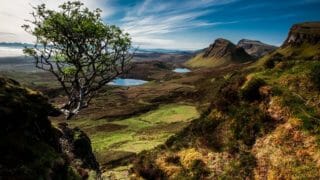
サンプルプログラムの作成
作成したサンプルプログラムの構成は以下の通り。
なお、上記の赤枠は、前提条件のプログラムから変更したプログラムである。
build.gradleの内容は以下の通りで、「spring-boot-starter-security」をライブラリに追加している。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | plugins { id 'org.springframework.boot' version '2.1.7.RELEASE' id 'java' } apply plugin: 'io.spring.dependency-management' group = 'com.example' version = '0.0.1-SNAPSHOT' sourceCompatibility = '1.8' repositories { mavenCentral() } configurations { //log4j2を利用するため、Spring BootデフォルトのLogbackを利用しないよう設定 all*.exclude module : 'spring-boot-starter-logging' } dependencies { implementation 'org.springframework.boot:spring-boot-starter-thymeleaf' implementation 'org.springframework.boot:spring-boot-starter-web' testImplementation 'org.springframework.boot:spring-boot-starter-test' compileOnly 'org.projectlombok:lombok:1.18.10' annotationProcessor 'org.projectlombok:lombok:1.18.10' compile files('lib/ojdbc6.jar') implementation 'org.mybatis.spring.boot:mybatis-spring-boot-starter:2.1.1' compile group: 'org.springframework.data', name: 'spring-data-commons-core', version: '1.1.0.RELEASE' //log4j2を利用するための設定 compile group: 'org.apache.logging.log4j', name: 'log4j-api', version: '2.12.1' compile group: 'org.apache.logging.log4j', name: 'log4j-core', version: '2.12.1' //AOPを利用するための設定 compile group: 'org.aspectj', name: 'aspectjweaver', version: '1.6.10' //log4j2の設定でymlファイルを利用するための設定 compile group: 'com.fasterxml.jackson.dataformat', name: 'jackson-dataformat-yaml', version: '2.10.1' compile group: 'com.fasterxml.jackson.core', name: 'jackson-core', version: '2.10.1' compile group: 'com.fasterxml.jackson.core', name: 'jackson-databind', version: '2.10.1' //Apache Common JEXLを利用するための設定 compile group: 'org.apache.commons', name: 'commons-jexl3', version: '3.0' //Spring Securityの設定 implementation 'org.springframework.boot:spring-boot-starter-security' } |
なお、前提条件のプログラムからbuild.gradleのみを修正した場合、以下のユーザー認証画面が表示される。
また、application.ymlの内容は以下の通りで、「spring.security.user.name」にユーザー名を、「spring.security.user.password」にパスワードを指定している。ここで定義した、ユーザー名「user」・パスワード「pass」を指定した場合のみ、Basic認証のログインができるようになっている。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | server: port: 8084 spring: # DB接続情報 datasource: url: jdbc:oracle:thin:@localhost:1521:xe username: USER01 password: USER01 driverClassName: oracle.jdbc.driver.OracleDriver # SpringSecurityユーザー情報 security: user: name: user password: pass # 一覧画面で1ページに表示する行数 demo: list: pageSize: 2 |
さらに、以下のWebSecurityConfigurerAdapterクラスを継承したクラスで、Spring Securityの認証方法の定義を行っている。この設定で、初期表示画面を表示した場合に、Basic認証のログイン画面が表示されるようになっている。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | package com.example.demo; import org.springframework.context.annotation.Configuration; import org.springframework.security.config.annotation.web.builders.HttpSecurity; import org.springframework.security.config.annotation.web.builders.WebSecurity; import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity; import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter; import org.springframework.security.config.http.SessionCreationPolicy; import org.springframework.security.web.firewall.DefaultHttpFirewall; @Configuration @EnableWebSecurity public class DemoSecurityConfig extends WebSecurityConfigurerAdapter { @Override public void configure(WebSecurity web) { //org.springframework.security.web.firewall.RequestRejectedException: //The request was rejected because the URL contained a potentially //malicious String ";"というエラーログがコンソールに出力されるため、下記を追加 DefaultHttpFirewall firewall = new DefaultHttpFirewall(); web.httpFirewall(firewall); } /** * SpringSecurityによる認証を設定 * @param http HttpSecurityオブジェクト * @throws Exception 例外 */ @Override protected void configure(HttpSecurity http) throws Exception { //初期表示画面を表示する際にBasic認証を実施する http.antMatcher("/").httpBasic() .and() //かつ //それ以外の画面は全て認証を有効にする .authorizeRequests().anyRequest().authenticated() .and() //かつ //リクエスト毎に認証を実施するようにする .sessionManagement().sessionCreationPolicy( SessionCreationPolicy.STATELESS); } } |
その他のソースコード内容は、以下のサイトを参照のこと。
https://github.com/purin-it/java/tree/master/spring-boot-security-basic/demo
サンプルプログラムの実行結果
サンプルプログラムの実行結果は、以下の通り。
1) Spring Bootアプリケーションを起動し、「http:// (ホスト名):(ポート番号)」とアクセスすると、以下のBasic認証画面が表示される
2) application.ymlに定義したユーザーと違うユーザー名またはパスワードを入力し、「OK」ボタンを押下
3) 以下のように、ログインができず、再度Basic認証の画面が表示されることが確認できる
4) application.ymlに定義したユーザーと同じユーザー名またはパスワードを入力し、「OK」ボタンを押下
5) 以下のように、ログインでき、検索画面が表示されることが確認できるので、「閉じる」ボタンを押下
6) 画面を閉じてよいかの確認画面が表示されるので、「はい」ボタンを押下
7) 「http:// (ホスト名):(ポート番号)」とアクセスすると、再度Basic認証画面が表示される
要点まとめ
- Spring Securityには、セキュリティ対策の基本機能として「認証」「認可」機能が含まれる。
- Spring Securityを利用するには、「spring-boot-starter-security」のライブラリを追加し、WebSecurityConfigurerAdapterクラスを継承したクラスで、Spring Securityの認証方法の定義を行う。
- Spring Securityの認証で、固定のユーザー・パスワードの場合のみ認証できるようにするには、application.yml(またはapplication.properties)の「spring.security.user.name」「spring.security.user.password」を指定する。